//**************************************神吹表格操作函数*******************************************************
//隐藏列
function setHiddenRow(tb,iCol){
for (i=0;i<oTable.rows.length;i++){
tb.rows[i].cells[iCol].style.display = oTable.rows[i].cells[iCol].style.display=="none"?"block":"none";
}
}
//隐藏行
function setHiddenRow(tb,iRow){
tb.rows[iRow].style.display = oTable.rows[iRow].style.display == "none"?"block":"none";
}
//创建表格
function createTable(id,rows,cells,tbid){
var tb=document.createElement("table");
var tbody = document.createElement("tbody");
for(var i=0;i<rows;i++){
var tr=document.createElement("tr");
for(var j=0;j<cells;j++){
var cell=document.createElement("td");
tr.appendChild(cell);
}
tbody.appendChild(tr);
}
tb.appendChild(tbody);
tb.setAttribute("id",tbid);//设置创建的TABLE的ID
document.getElementById(id).appendChild(tb);
}
//插入文本
function insertText(tb,row,cel,text){
txt=document.createTextNode(text);
tb.rows[row].cells[cel].appendChild(txt);
}
//修改文本
function updateText(tb,row,cel,text){
tb.rows[row].cells[cel].firstChild.nodeValue=text;
}
//添加子节点
function toAppendChild(tb,row,cel,child){
tb.rows[row].cells[cel].appendChild(child);
}
//删除某行
function removeRow(tb,row){
tb.lastChild.removeChild(tb.rows[row]);
}
//单元格设置属性
function cellSetAttribute(tb,row,col,attributeName,attributeValue){
tb.rows[row].cells[col].setAttribute(attributeName,attributeValue);
}
//单元格添加监听器
function cellAddListener(tb,row,cel,event,fun){
if(window.addEventListener)
{
//其它浏览器的事件代码: Mozilla, Netscape, Firefox
//添加的事件的顺序即执行顺序 //注意用 addEventListener 添加带on的事件,不用加on
// img.addEventListener('click', delRow(this), true);
tb.rows[row].cells[cel].addEventListener(event,fun, true);
}
else
{
//IE 的事件代码 在原先事件上添加 add 方法
// img.attachEvent('onclick',delRow(this));
tb.rows[row].cells[cel].attachEvent("on"+event,fun);
}
}
//新增行
function insertRow(oTable){
var tr=document.createElement("tr");
for (i=0;i<oTable.rows[0].cells.length;i++){
var td= document.createElement("td");
tr.appendChild(td);
}
oTable.lastChild.appendChild(tr);
}
//行设置属性
function rowSetAttribute(tb,row,attributeName,attributeValue){
tb.rows[row].setAttribute(attributeName,attributeValue);
}
//行添加监听器
function rowAddListener(tb,row,event,fun){
if(window.addEventListener)
{
//其它浏览器的事件代码: Mozilla, Netscape, Firefox
//添加的事件的顺序即执行顺序 //注意用 addEventListener 添加带on的事件,不用加on
// img.addEventListener('click', delRow(this), true);
tb.rows[row].addEventListener(event,fun, true);
}
else
{
//IE 的事件代码 在原先事件上添加 add 方法
// img.attachEvent('onclick',delRow(this));
tb.rows[row].attachEvent("on"+event,fun);
}
}
//新增列
function addCells(tb){
for (i=0;i<tb.rows.length;i++){
var td= document.createElement("td");
tb.rows[i].appendChild(td);
}
}
//批量修改单元格属性
function cellsSetAttribute(oTable,attributeName,attributeValue){
for (i=0;i<oTable.rows.length;i++){
for (j=0;j<oTable.rows[i].cells.length;j++){
oTable.rows[i].cells[j].setAttribute(attributeName,attributeValue);
}
}
}
//合并只支持单向合并
//行合并
function mergerRow(tb,row,cell,num){
for(var i= (row+1),j=0;j<(num-1);j++){
tb.rows[i].removeChild(tb.rows[i].cells[cell]);
}
tb.rows[row].cells[cell].setAttribute("rowspan",num);
// document.getElementById('c').innerHTML=document.getElementById('u').innerHTML;
}
//列合并
function mergerCell(tb,row,cell,num){
for(var i= (cell+1), j=0;j<(num-1);j++){
tb.rows[row].removeChild(tb.rows[row].cells[i]);
}
tb.rows[row].cells[cell].setAttribute("colspan",num);
// document.getElementById('c').innerHTML=document.getElementById('u').innerHTML;
}
测试DEMO
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title> new document </title>
<meta name="generator" content="editplus" />
<meta name="author" content="" />
<meta name="keywords" content="" />
<meta name="description" content="" />
<style>
.testclass{background-color:yellow;}
</style>
<script type="text/javascript" src="stone.js"></script>
<script type="text/javascript">
<!--
function giveText(){
for(var i=0;i<5;i++){
for(var j=0;j<5;j++){
insertText(mytable,i,j,i*5+j);
}
}
}
function addInput(){
var input = document.createElement("input");
input.setAttribute("type","text");
input.setAttribute("value","我是新增的");
toAppendChild(mytable,3,3,input);
}
function listen(){
alert('恭喜你!监听器安装成功!');
}
//-->
</script>
</head>
<body>
表格函数测试<br />
<div id="u">
</div>
<input type="button" value="新建一个5X5的表格" onclick="createTable('u',5,5,'mytable');" />
<input type="button" value="显示表格边框" onclick="document.getElementById('mytable').setAttribute('border',1);" />
<input type="button" value="插入文本" onclick="giveText();" />
<input type="button" value="修改文本" onclick="updateText(mytable,3,3,'text')" /> <br />
<input type="button" value="添加子节点input" onclick="addInput();" />
<input type="button" value="删除第5行" onclick="removeRow(mytable,4);" />
<input type="button" value="设置单元格宽度" onclick="cellSetAttribute(mytable,0,0,'width','50')" />
<input type="button" value="添加单元格监听器" onclick="cellAddListener(mytable,2,2,'click',listen)" /> <br />
<input type="button" value="行合并" onclick="mergerRow(mytable,2,1,2); document.getElementById('u').innerHTML=document.getElementById('u').innerHTML;" />
<input type="button" value="列合并" onclick="mergerCell(mytable,1,2,3); document.getElementById('u').innerHTML=document.getElementById('u').innerHTML;" />
<input type="button" value="设置单元格背景色" onclick="cellsSetAttribute(mytable,'class','testclass'); document.getElementById('u').innerHTML=document.getElementById('u').innerHTML;" />
<input type="button" value="设置行高" onclick="rowSetAttribute(mytable,2,'height','50');" /> <br />
<input type="button" value="新增第4行监听器" onclick="rowAddListener(mytable,3,'click',listen);" />
<input type="button" value="新增一行" onclick="insertRow(mytable);" />
<input type="button" value="新增列" onclick="addCells(mytable);" />
</body>
</html>
测试截图:
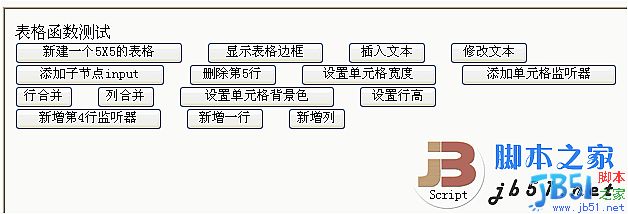
以上就是【一组JS创建和操作表格的函数集合】的全部内容了,欢迎留言评论进行交流!